An Azure Logic App can be used to schedule work by calling an uri at a specified time during a period or in intervals during a period. It is easy to add header values and query parameters in a HTTP-action, it is not as easy to send multipart form-data.
Logic App
Create a Logic App in Azure Portal, add a Schedule-action and a HTTP-action in the Design view. You can select POST as the method, add the URI and save while your are in the Design view.
Postman
Open Postman and create a new request. Add all the form-data that is needed for the request, click on the Code link and select “Java OK HTTP”. The output includes a Content-Type header value and body contents.
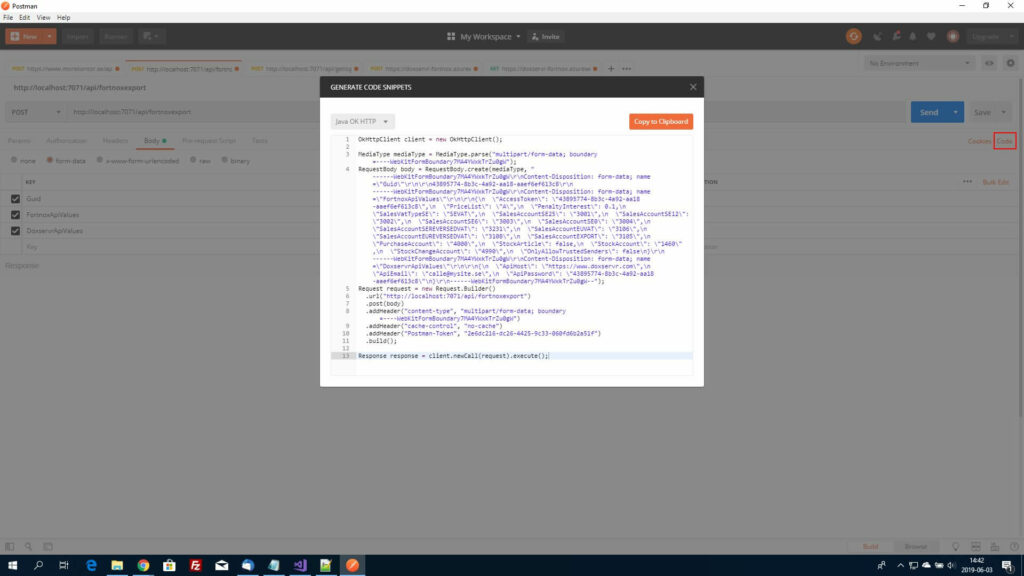
OkHttpClient client = new OkHttpClient();
MediaType mediaType = MediaType.parse("multipart/form-data; boundary=----WebKitFormBoundary7MA4YWxkTrZu0gW");
RequestBody body = RequestBody.create(mediaType, "------WebKitFormBoundary7MA4YWxkTrZu0gW\r\nContent-Disposition: form-data; name=\"Guid\"\r\n\r\n43895774-8b3c-4a92-aa18-aaef6ef613c8\r\n------WebKitFormBoundary7MA4YWxkTrZu0gW\r\nContent-Disposition: form-data; name=\"FortnoxApiValues\"\r\n\r\n{\n \"AccessToken\": \"43895774-8b3c-4a92-aa18-aaef6ef613c8\",\n \"PriceList\": \"A\",\n \"PenaltyInterest\": 0.1,\n \"SalesVatTypeSE\": \"SEVAT\",\n \"SalesAccountSE25\": \"3001\",\n \"SalesAccountSE12\": \"3002\",\n \"SalesAccountSE6\": \"3003\",\n \"SalesAccountSE0\": \"3004\",\n \"SalesAccountSEREVERSEDVAT\": \"3231\",\n \"SalesAccountEUVAT\": \"3106\",\n \"SalesAccountEUREVERSEDVAT\": \"3108\",\n \"SalesAccountEXPORT\": \"3105\",\n \"PurchaseAccount\": \"4000\",\n \"StockArticle\": false,\n \"StockAccount\": \"1460\",\n \"StockChangeAccount\": \"4990\",\n \"OnlyAllowTrustedSenders\": false\n}\r\n------WebKitFormBoundary7MA4YWxkTrZu0gW\r\nContent-Disposition: form-data; name=\"DoxservrApiValues\"\r\n\r\n{\n \"ApiHost\": \"https://www.doxservr.com\",\n \"ApiEmail\": \"calle@mysite.se\",\n \"ApiPassword\": \"43895774-8b3c-4a92-aa18-aaef6ef613c8\"\n}\r\n------WebKitFormBoundary7MA4YWxkTrZu0gW--");
Request request = new Request.Builder()
.url("http://localhost:7071/api/fortnoxexport")
.post(body)
.addHeader("content-type", "multipart/form-data; boundary=----WebKitFormBoundary7MA4YWxkTrZu0gW")
.addHeader("cache-control", "no-cache")
.addHeader("Postman-Token", "1656e641-082a-486a-9a0f-9b9273117641")
.build();
Response response = client.newCall(request).execute();
Add header and body content
Open the Code view for your logic app and add a Content-Type header according to the output from Postman and replace the body contents with the string output from Postman.
{
"definition": {
"$schema": "https://schema.management.azure.com/providers/Microsoft.Logic/schemas/2016-06-01/workflowdefinition.json#",
"actions": {
"HTTP": {
"inputs": {
"body": "------WebKitFormBoundary7MA4YWxkTrZu0gW\r\nContent-Disposition: form-data; name=\"Guid\"\r\n\r\n@{guid()}\r\n------WebKitFormBoundary7MA4YWxkTrZu0gW\r\nContent-Disposition: form-data; name=\"FortnoxApiValues\"\r\n\r\n{\n \"AccessToken\": \"43895774-8b3c-4a92-aa18-aaef6ef613c8\",\n \"PriceList\": \"A\",\n \"PenaltyInterest\": 0.1,\n \"SalesVatTypeSE\": \"SEVAT\",\n \"SalesAccountSE25\": \"3001\",\n \"SalesAccountSE12\": \"3002\",\n \"SalesAccountSE6\": \"3003\",\n \"SalesAccountSE0\": \"3004\",\n \"SalesAccountSEREVERSEDVAT\": \"3231\",\n \"SalesAccountEUVAT\": \"3106\",\n \"SalesAccountEUREVERSEDVAT\": \"3108\",\n \"SalesAccountEXPORT\": \"3105\",\n \"PurchaseAccount\": \"4000\",\n \"StockArticle\": false,\n \"StockAccount\": \"1460\",\n \"StockChangeAccount\": \"4990\",\n \"OnlyAllowTrustedSenders\": false\n}\r\n------WebKitFormBoundary7MA4YWxkTrZu0gW\r\nContent-Disposition: form-data; name=\"DoxservrApiValues\"\r\n\r\n{\n \"ApiHost\": \"https://www.doxservr.com\",\n \"ApiEmail\": \"calle@mysite.se\",\n \"ApiPassword\": \"43895774-8b3c-4a92-aa18-aaef6ef613c8\"\n}\r\n------WebKitFormBoundary7MA4YWxkTrZu0gW--",
"headers": {
"Content-Type": "multipart/form-data; boundary=----WebKitFormBoundary7MA4YWxkTrZu0gW"
},
"method": "POST",
"uri": "https://mysite.azurewebsites.net/api/function"
},
"runAfter": {},
"type": "Http"
}
},
"contentVersion": "1.0.0.0",
"outputs": {},
"parameters": {},
"triggers": {
"Återkommande": {
"recurrence": {
"frequency": "Day",
"interval": 1,
"schedule": {
"hours": [
"0"
]
},
"timeZone": "UTC"
},
"type": "Recurrence"
}
}
}
}
I you need to use expressions in the request like @{guid()} in this example, replace values with expressions. Save the logic app and test it. Do not go to Design view and save the application, this can mess up the encoding of body contents.
Thanks for sharing that, it really helped me !
There best suitable output I found in Postman is the NodeJs – Native code snippet, I just had to change the boundary part in Content-Type header to make it work
Thanks, it worked for me.
Just replace the values in body and go